Why Use React: An Effective Web Development Tool
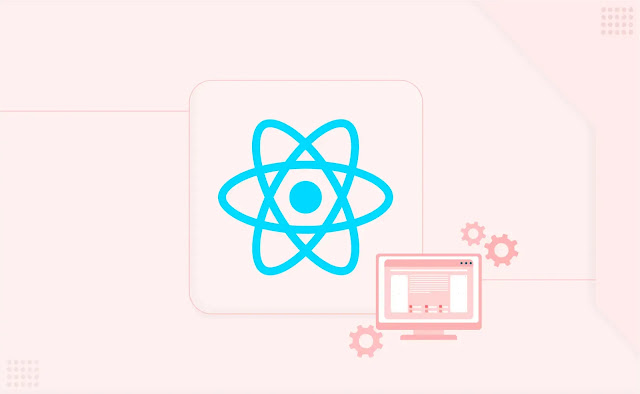
A leading software development company specializing in web and mobile app solutions. We started our journey in 2011 and since then it has been quite a success. We believe in consistently driving and delivering innovation. We prioritize delivering software products that address your unique business needs. Our software product engineering process is designed to provide a seamless and collaborative experience.
Introduction
Unit testing is key to modern web development, and code reliability and maintainability. In the React world, Jest and Enzyme are the two tools that make testing components and logic a breeze. This post will go over unit testing in React with Jest and Enzyme, a complete guide to help you get up to speed with these tools and speed up your development.
Unit testing checks that individual pieces of an application work as expected. In React terms, that means testing components, functions and hooks to make sure they work in isolation. Here’s why unit testing is important:
Jest is a JavaScript testing framework by Facebook. Simple, powerful and works well with React.
Enzyme is a JavaScript testing utility for React by Airbnb. Makes it easier to manipulate, traverse and assert components.
First, you have a React project. If not, create one using Create React App:
bashCopy codenpx create-react-app my-app
cd my-app
Next, install Jest and Enzyme:
bashCopy codenpm install --save-dev jest enzyme enzyme-adapter-react-16
Configure Enzyme to work with Jest by creating a setup file:
javascriptCopy code// src/setupTests.js
import { configure } from 'enzyme';
import Adapter from 'enzyme-adapter-react-16';
configure({ adapter: new Adapter() });
Update your package.json
to include the setup file:
jsonCopy code"jest": {
"setupFilesAfterEnv": ["<rootDir>/src/setupTests.js"]
}
Let's write a simple test for a React component. Consider the following Button
component:
javascriptCopy code// src/components/Button.js
import React from 'react';
const Button = ({ label }) => <button>{label}</button>;
export default Button;
Create a test file for the Button
component:
javascriptCopy code// src/components/Button.test.js
import React from 'react';
import { shallow } from 'enzyme';
import Button from './Button';
describe('Button component', () => {
it('should render correctly with given label', () => {
const wrapper = shallow(<Button label="Click me" />);
expect(wrapper.text()).toBe('Click me');
});
});
Run your tests using:
bashCopy codenpm test
Jest is a testing framework that provides a full testing environment, test runner, assertion library and mocking capabilities. Enzyme is a utility that works alongside Jest to provide a more intuitive API for testing React components, to do shallow, mount and render testing.
Jest can handle most of the testing, but Enzyme provides advanced features for testing React components, like shallow rendering, that allows you to test components in isolation without rendering child components.
Jest provides a built-in mocking system. You can mock functions using jest.fn()
or jest.mock()
. Here's an example:
javascriptCopy codeconst mockFunction = jest.fn();
mockFunction();
expect(mockFunction).toHaveBeenCalled();
Yes, Jest supports testing asynchronous code. You can use async/await
, done
callbacks, or return promises. Here's an example:
javascriptCopy codeit('should fetch data successfully', async () => {
const data = await fetchData();
expect(data).toEqual(mockData);
});
Jest includes built-in support for snapshot testing. This captures the rendered output of a component and compares it to a saved snapshot. Here's how to use it:
javascriptCopy codeimport React from 'react';
import renderer from 'react-test-renderer';
import Button from './Button';
it('renders correctly', () => {
const tree = renderer.create(<Button label="Snapshot" />).toJSON();
expect(tree).toMatchSnapshot();
});
React hooks require special handling in tests. Use the react-hooks-testing-library
for this purpose:
bashCopy codenpm install --save-dev @testing-library/react-hooks
Here's an example of testing a custom hook:
javascriptCopy codeimport { renderHook, act } from '@testing-library/react-hooks';
import useCustomHook from './useCustomHook';
describe('useCustomHook', () => {
it('should increment counter', () => {
const { result } = renderHook(() => useCustomHook());
act(() => {
result.current.increment();
});
expect(result.current.count).toBe(1);
});
});
Mock API calls to test how your components handle data fetching:
javascriptCopy codeimport axios from 'axios';
import MockAdapter from 'axios-mock-adapter';
const mock = new MockAdapter(axios);
mock.onGet('/users').reply(200, [{ id: 1, name: 'John Doe' }]);
// Now you can test your component that makes API calls
Jest can be used to measure performance by checking execution time:
javascriptCopy codeit('should be performant', () => {
const start = performance.now();
render(<Component />);
const end = performance.now();
expect(end - start).toBeLessThan(50);
});
Unit testing in React with Jest and Enzyme is a powerful combination that ensures your components and logic are robust, reliable, and maintainable. By integrating these frameworks into your development process, you can catch bugs early, facilitate refactoring, and maintain high code quality. Start implementing these strategies today to elevate your React development workflow.
Comments
Post a Comment