Why Use React: An Effective Web Development Tool
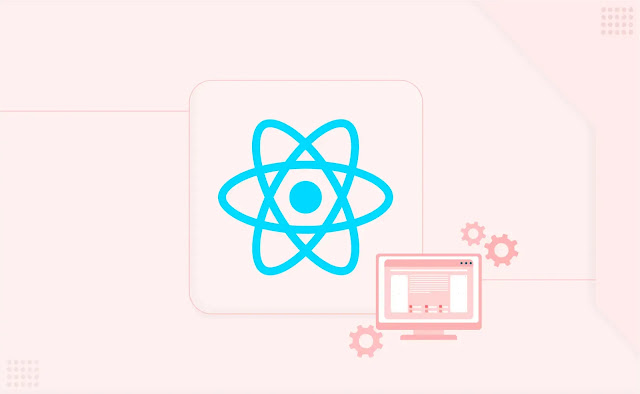
A leading software development company specializing in web and mobile app solutions. We started our journey in 2011 and since then it has been quite a success. We believe in consistently driving and delivering innovation. We prioritize delivering software products that address your unique business needs. Our software product engineering process is designed to provide a seamless and collaborative experience.
End-to-End (E2E) testing is a critical component of ensuring the functionality and reliability of your Angular applications. By testing the entire application workflow from start to finish, you can guarantee that all parts work together seamlessly. This guide focuses on using Cypress, a modern and developer-friendly E2E testing framework, for Angular applications.
E2E testing evaluates the complete flow of your application, covering user interactions, navigation, and data validation. It ensures that all components, services, and interactions function correctly as a whole. This comprehensive testing approach is essential for delivering high-quality applications.
Traditionally, Angular developers have used Protractor for E2E testing. However, Protractor has been deprecated, and many developers are now switching to Cypress due to its modern features and ease of use. Cypress offers fast test execution, automatic waiting, and excellent debugging tools, making it a superior choice for E2E testing.
First, install Cypress in your Angular project:
bashnpm install cypress --save-dev
Add Cypress commands to your package.json
:
json"scripts": {
"cypress:open": "cypress open",
"cypress:run": "cypress run"
}
Open Cypress to create the necessary configuration files:
bashnpm run cypress:open
This will create a cypress
folder with the following structure:
cypress ├── fixtures ├── integration ├── plugins └── support cypress.json
Modify cypress.json
to set up your base URL and other configurations:
json{
"baseUrl": "http://localhost:4200",
"integrationFolder": "cypress/integration",
"fixturesFolder": "cypress/fixtures",
"pluginsFile": "cypress/plugins/index.js",
"supportFile": "cypress/support/index.js"
}
Create a test file in the cypress/integration
folder, for example, home.spec.js
:
javascriptdescribe('Home Page', () => {
it('should display the welcome message', () => {
cy.visit('/');
cy.contains('Welcome to Your Angular App').should('be.visible');
});
});
Focus on the most common and important user scenarios rather than testing every possible edge case. This approach saves time and resources while ensuring critical functionality works as expected.
Prioritize business-impacted features before less important edge cases. This ensures that the most crucial parts of your application are tested thoroughly.
Simulate real-life user interactions as much as possible. For example, mimic actions such as looking at images or watching videos before proceeding with other actions.
Reduce the complexity of E2E testing by resolving errors during coding before running the tests. This practice helps streamline the testing process.
Create an optimal test environment to minimize setup time and clear out data for subsequent tests. This ensures consistent and reliable test results.
Ensure your E2E tests can handle authentication if your Angular application requires user login. Use test users or tokens to authenticate during tests.
Isolate your E2E tests from external dependencies by mocking backend services. Tools like ng-mocks, Angular’s HttpClientTestingModule, or interceptors can be used to stub API calls and responses.
Integrate Cypress tests with your CI/CD pipeline to catch regressions early. Here’s an example configuration for GitHub Actions:
yamlname: CI
on: [push, pull_request]
jobs:
e2e-tests:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Node.js
uses: actions/setup-node@v2
with:
node-version: '14'
- run: npm install
- run: npm run start &
continue-on-error: true
- run: npx wait-on http://localhost:4200
- run: npm run cypress:run
E2E testing is essential for ensuring the reliability and functionality of your Angular applications. Cypress, with its modern features and ease of use, is an excellent choice for E2E testing. By following the steps and best practices outlined in this guide, you can effectively set up and execute E2E tests, providing confidence that your application behaves as expected.
Boost your Angular application’s reliability with comprehensive end-to-end testing. Discover expert tips and strategies for seamless Angular E2E testing. Start improving your workflow today!
Comments
Post a Comment