Why Use React: An Effective Web Development Tool
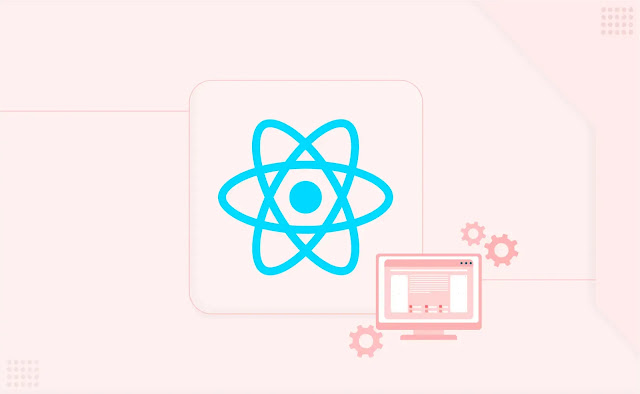
A leading software development company specializing in web and mobile app solutions. We started our journey in 2011 and since then it has been quite a success. We believe in consistently driving and delivering innovation. We prioritize delivering software products that address your unique business needs. Our software product engineering process is designed to provide a seamless and collaborative experience.
Functional components in React are great for building UIs declaratively, but sometimes you need to interact directly with the DOM. This is where the useRef
hook comes in. Introduced in React 16.8, useRef
helps manage DOM elements and mutable values without causing re-renders.
Why Use useRef
?
useRef
to directly manipulate DOM elements.useState
, updating useRef
doesn't cause re-renders.How useRef
Works
The useRef
hook returns a mutable object with a current
property. This property can hold any value and won't change unless explicitly updated. This makes useRef
perfect for storing values that need to persist between renders, like input field values.
Example Usage
javascriptimport React, { useRef } from 'react';
function MyComponent() {
const myRef = useRef(true);
console.log(myRef.current); // true
return (
<div>
<input ref={myRef} />
</div>
);
}
export default MyComponent;
Advantages of useRef
In conclusion, the useRef
hook is a powerful tool in React for managing DOM elements and persistent values efficiently, enhancing your application's performance and usability.
Need skilled and dedicated Angular developers for your next project? Hire the best from AngularMinds Pvt Ltd to bring your vision to life with expertise and precision. Contact us today!
Comments
Post a Comment